Adding Folder
Here, we created a folder in our project to store uploaded files.
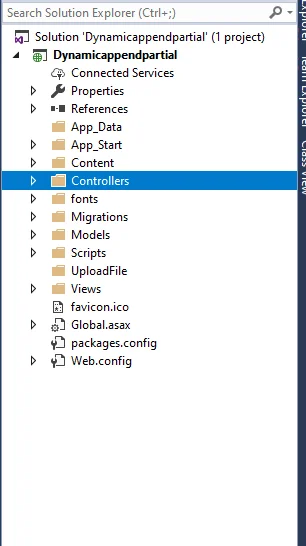
[Fig :- Storing Uploaded File]
We need to create a model class for file upload and return files. Right-click on Model Folder and add a class file. Here I will use the code-first approach to implement File upload and return functionality. Place the following code inside the class file.
Filetables.cs
using System.Collections.Generic;
using System.Web;
namespace Dynamicappendpartial.Models
{
public class Filetables
{
public IEnumerable files { get; set; }
public int Id { get; set; }
public string File { get; set; }
public string Type { get; set; }
}
}
Right-click on Models and add Ado.Net Entity Data model.
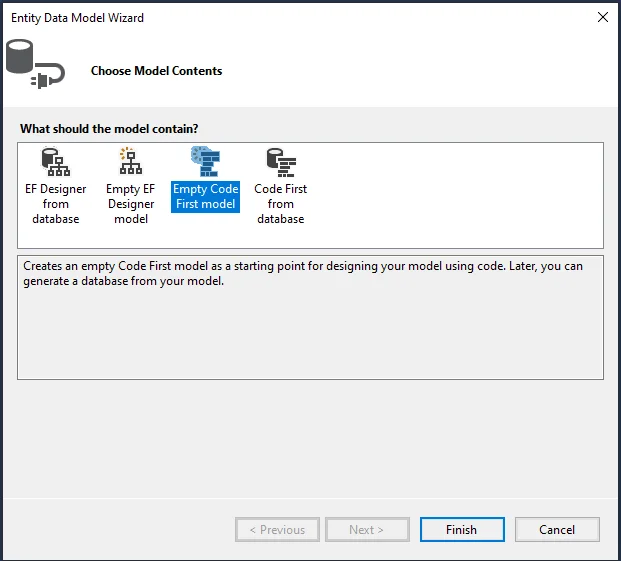
[Fig :- Empty Code First model]
This Empty code First model creates a context file for your database connection. You can now add your Filetables class within the context file shown below.
using System;
using System.Data.Entity;
using System. Linq;
namespace Dynamicappendpartial.Models
{
public class FileBlog: DbContext
{
public FileBlog(): base("name=FileBlog")
{
}
public virtual DbSet Filetables { get; set; }
}
}
Now, we need to execute all the required Migrations commands within the package manager control.
We need to add a controller. Right-click on the Controller folder and click on add > controller.
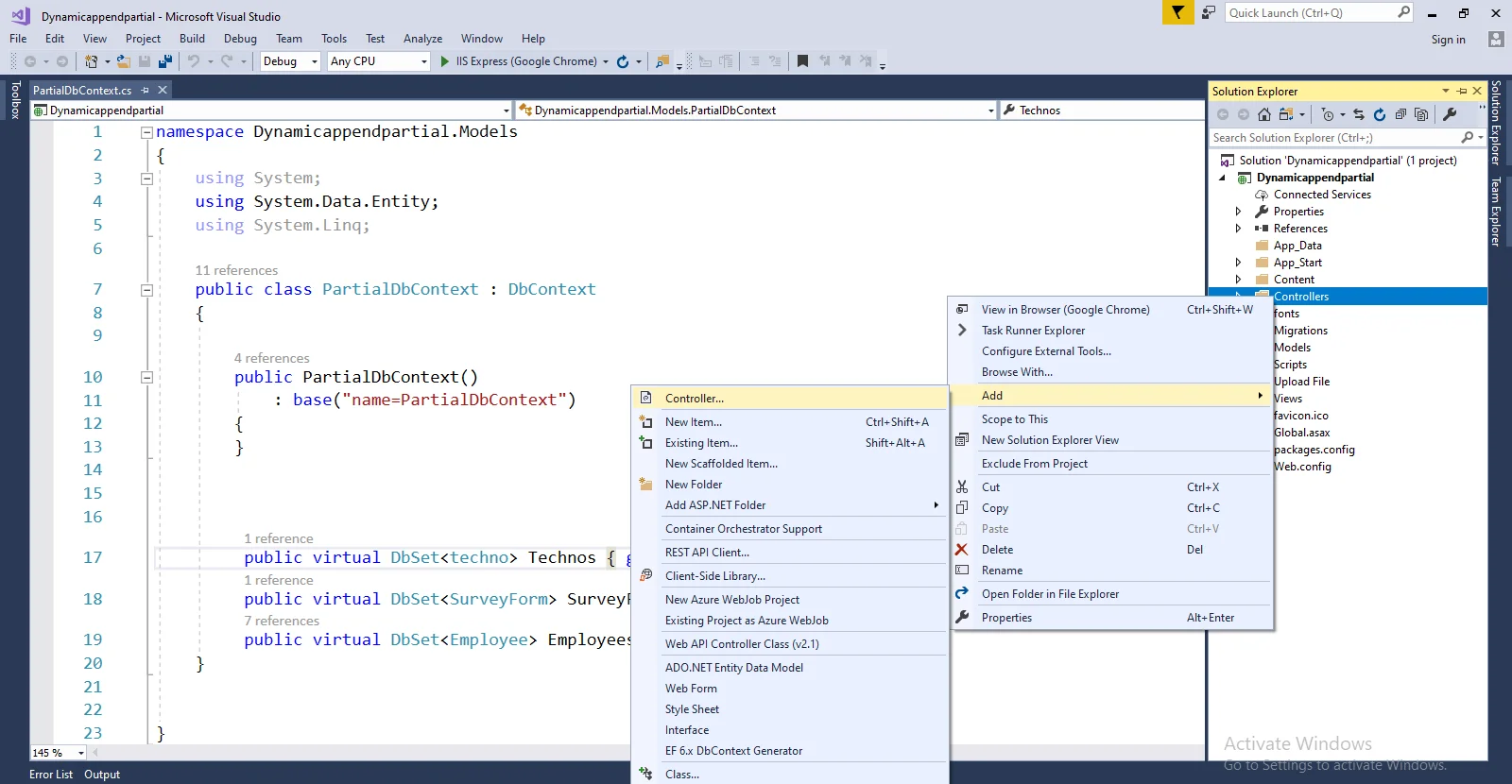
[Fig :- Creating Controller]
Select empty controller form list and give the suitable name of the controller. Here I give controller name as Filetables. And place the following code inside the controller.
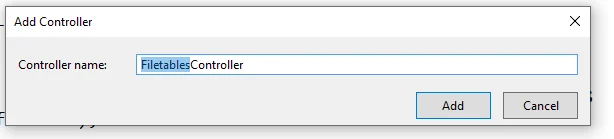
[Fig :- Add Controller]
FiletableController
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.IO;
using System.Linq;
using System.Net;
using System.Web;
using System.Web.Mvc;
using Dynamicappendpartial.Models;
namespace Dynamicappendpartial.Controllers
{
public class FiletablesController : Controller
{
private FileBlog db = new FileBlog();
// GET: Filetables
public ActionResult Index()
{
List ObjFiles = new List();
foreach (string strfile inDirectory.GetFiles(Server.MapPath("~/UploadFile")))
{
FileInfo fi = new FileInfo(strfile);
Filetables obj = new Filetables();
obj.File = fi.Name;
obj.Type = GetFileTypeByExtension(fi.Extension);
ObjFiles.Add(obj);
}
return View(ObjFiles);
}
public FileResult Download(string fileName)
{
string fullPath = Path.Combine(Server.MapPath("~/UploadFile"), fileName);
byte[] fileBytes = System.IO.File.ReadAllBytes(fullPath);
return File(fullPath, "application/force-download", Path.GetFileName(fullPath));
}
private string GetFileTypeByExtension(string extension)
{
switch (extension.ToLower())
{
case ".docx":
case ".doc":
return "Microsoft Word Document";
case ".xlsx":
case ".xls":
return "Microsoft Excel Document";
case ".txt":
return "Text Document";
case ".jpg":
case ".png":
return "Image";
default:
return "Unknown";
}
}
[HttpPost]
public ActionResult Index(Filetables doc)
{
try
{
foreach (var file in doc.files)
{
if (file.ContentLength > 0)
{
var fileName = Path.GetFileName(file.FileName);
var filePath = Path.Combine(Server.MapPath("~/UploadFile"), fileName);
file.SaveAs(filePath);
}
}
TempData["Message"] = "files uploaded successfully";
return RedirectToAction("Index");
}
catch
{
TempData["Message"] = "File upload Failed!!";
return RedirectToAction("Index");
}
}
}
}
Here I am creating two index methods one for uploading content to the database and one for uploading the file. The first index method is used to retrieve the uploaded file list and display it on the index page. On the same index page, I will create a form to upload files in the database.
The Last Index method in the controller is for uploading a file on a server. I want to store all files inside the upload file folder we created before starting the code. And use the TempData to display the message after files are uploaded successfully. If any exception occurs during execution or if the file was not uploaded successfully TempData shows File Uploaded Failed message on view.
In the controller, I am creating one method for file Extension. This method is used to display which type of file we uploaded to the database. And also create a method for downloading an uploaded file.
Right-click on the index method and add a view for the index method.
IndexView
@model IEnumerable
@{
ViewBag.Title = "Index";
}
@using (@Html.BeginForm(null, null, FormMethod.Post,
new { enctype = "multipart/form-data" }))
{
if (TempData["Message"] != null)
{
@TempData["Message"]
}
}
@foreach (var item in Model)
{
}
Here in view I am creating an upload file and retrieve a list of the uploaded file in the same view you can modify the code as per your requirements.
For the Uploading file, we have to require an input type file so we can select the file.
Now run the application. On index view, you can show the choose file option.
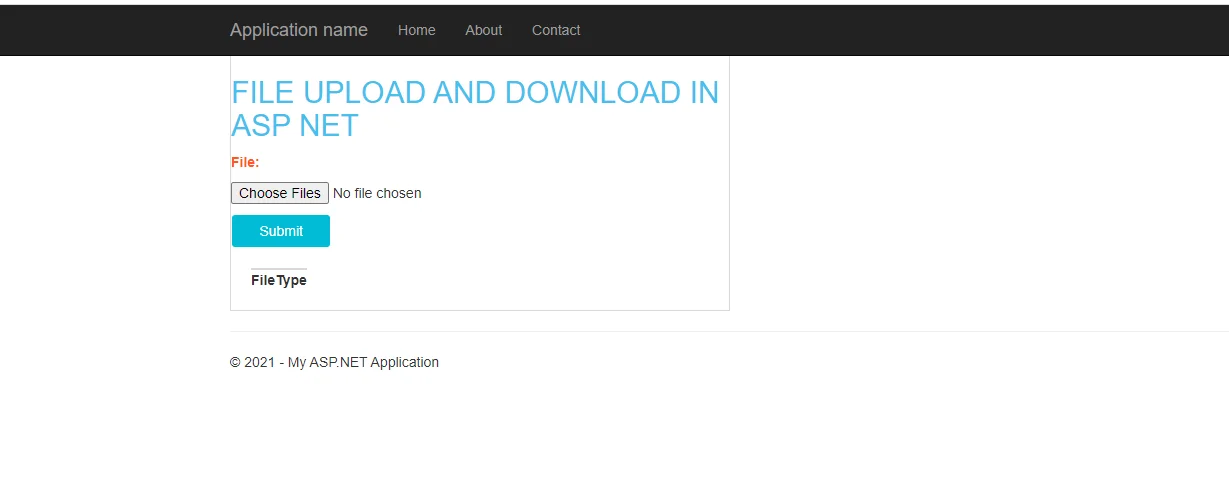
[Fig :- Index view for File Uploading]
If the file was upload successfully you can show the file upload successfully message in view.
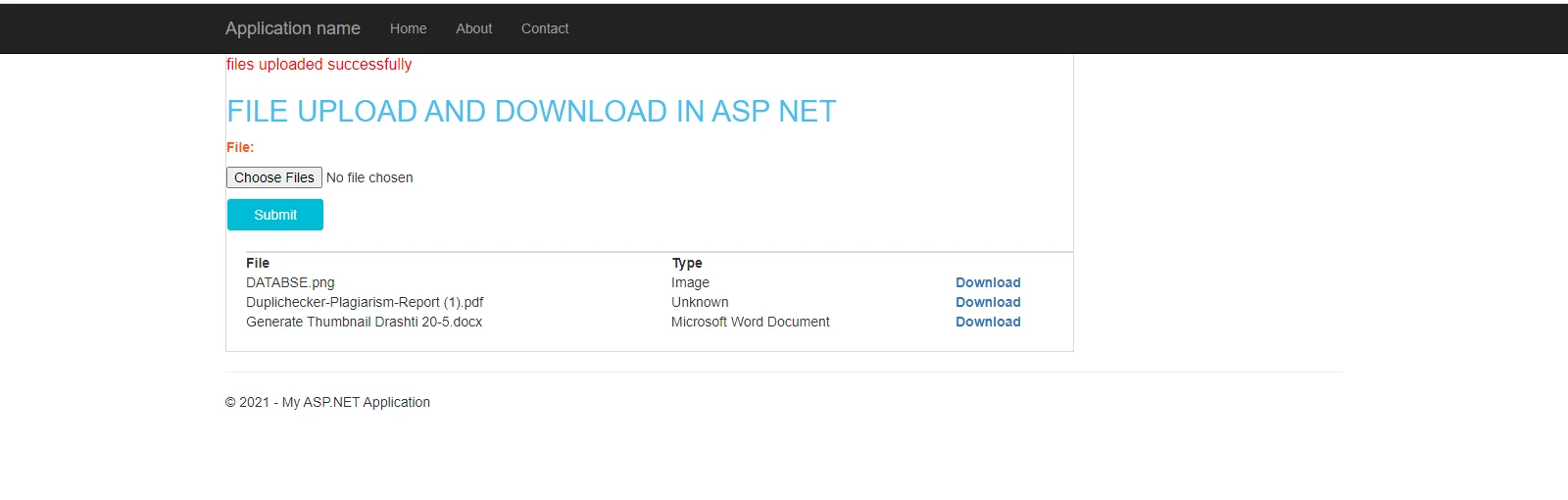
[Fig :- Uploaded file Display with the message]
If the file was not uploaded or we can click submit button without selecting the file it will display the File upload failed message in view.
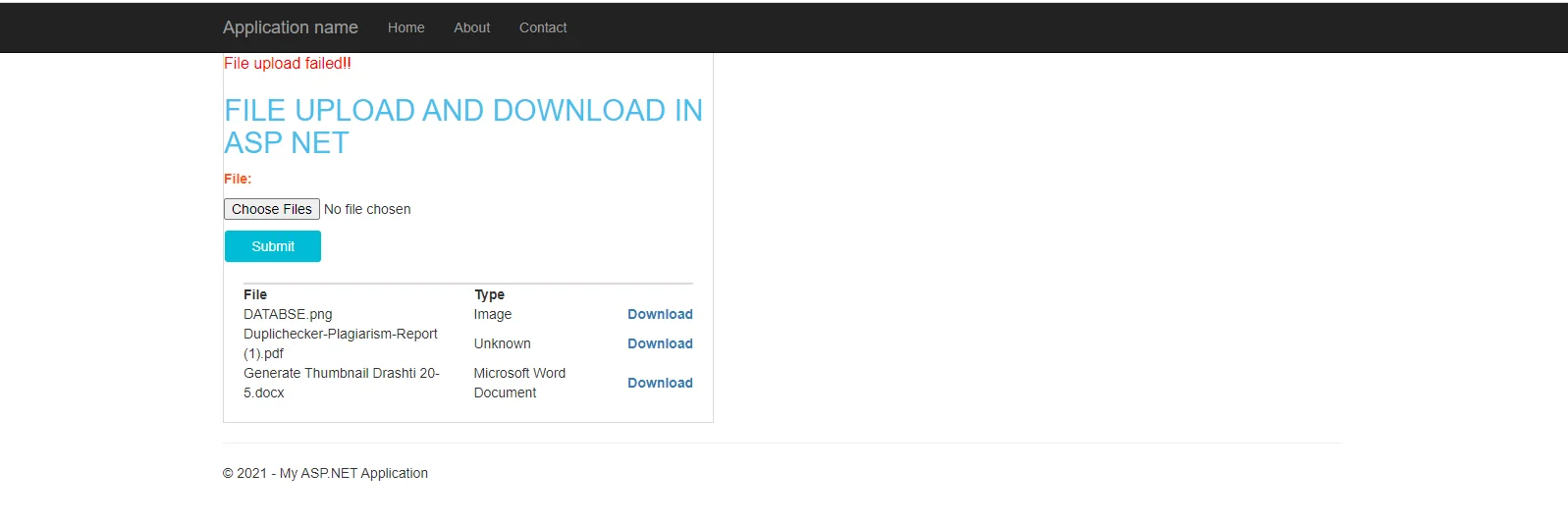
[Fig :- Uploaded file with the failed message]

[Fig :- Uploaded file]
You can see all the uploaded files inside the folder we have created.
Click on the Download link to download the file. Here I download all files you can show the downloaded file in the browser.
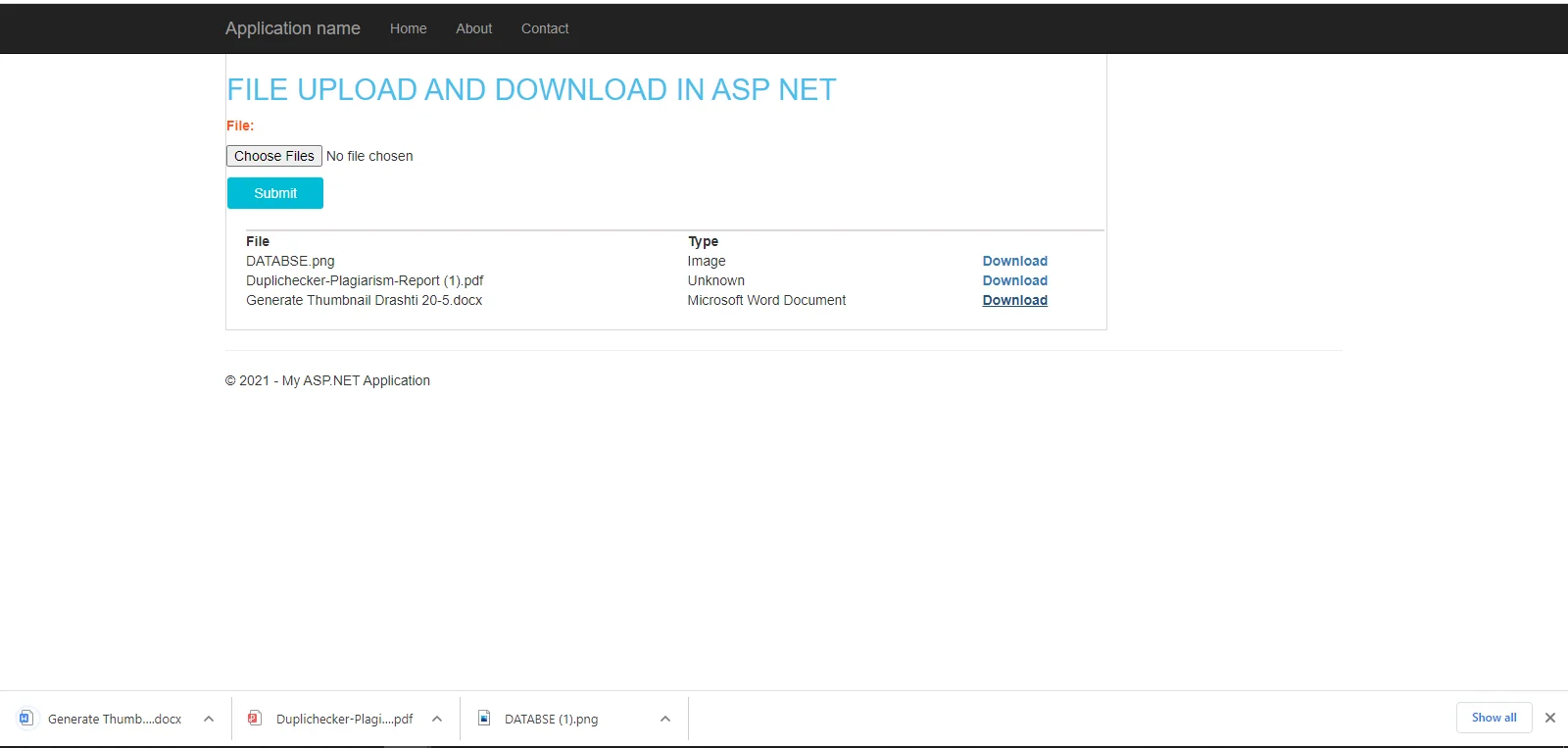
[Fig :- Download File]