Create a The NET Class Library Project
There are the following steps to add a new the .NET class library project named “MathLibrary” to the solution
-
You can Right-click on the solution in the Solution Explorer and select Add -> New Project.
-
In the Add a new project page, you can write the library in the search box. Select C# or Visual Basic from your language list, and select all platforms from your Platform list.
-
Select the .net Class Library template, and after you can select Next.
-
In the Configure your new project page, you can enter MathLibrary in the Project name box, and after you can select Next.
-
In the Additional information page, you can select .NET 5.0 (Current), and after choose to create.
Ensure that the library targets the correct version of .NET. You can Right-click on the class library project in Solution Explorer, and then choose Properties. The Target Framework text box displays that the project targets .NET 5.0.
When you’re using Visual Basic, you can clear the text in the Root namespace text box.
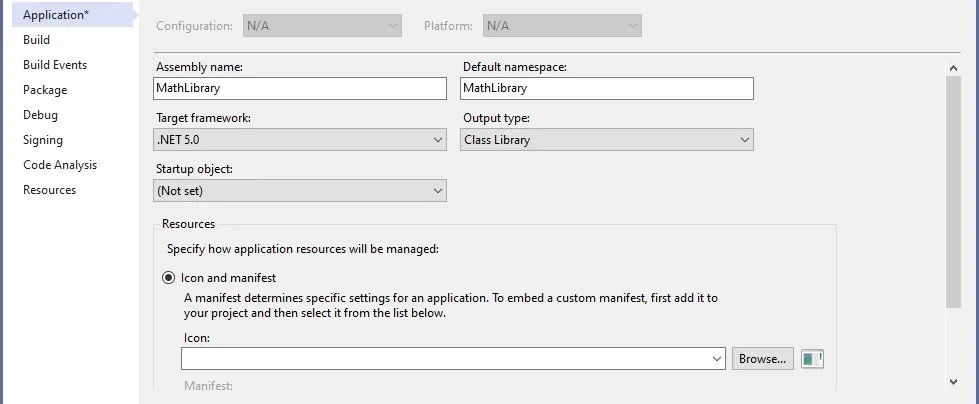
Figure: Property window of The .NET Class Library
For every project, Visual Basic automatically creates a namespace matching the name of the project.
using System;
namespace MathLibrary
{
public class MathOperation
{
public double Perform(double n1, double n2, string operation)
{
double data = 0;
switch(operation)
{
case "Addition":
data = n1 + n2;
break;
case "Subtraction":
data = n1 - n2;
break;
case "Multiplication":
data = n1 * n2;
break;
case "Division":
data = (double)n1 / n2;
break;
case "Modulo":
data = n1 % n2;
break;
default:
Console.WriteLine("Plz you check your some operatiom");
break;
}
return data;
}
}
}
First of all, we will rename the class1.cs file and change the name of MathOperation after containing a method name of Perform. In the Perform method, we can pass two operands and one string data type respectively n1 n2 and “operation”. The user can select any of the basic math operations such as Addition, Subtraction, Multiplication, Division, and Modulo so we can pass the “operation” value in the switch case, so a user can perform any of the math operations. If the user selects the wrong operation so we can pass the “default” value.
In the Menu bar, you can select the Build -> Build Solution or press Ctrl + Shift + B to verify that the project compiles without error.
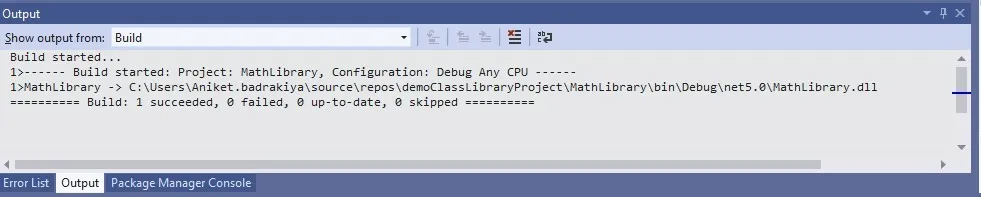
Figure: Output window of The .Net Class Library