To create ASP.NET Core Console Application follow the below steps:
Step 1:Open Visual Studio, select File option -> New option -> Project
Step 2:elect the ASP.NET Core Console Application template and click Next.
Step 3:Enter the project name, and then click Create.
Figure 1 Create an application
Step 4:Create a new class
Add new class Calculator.cs
namespace UniTestDemo
{
public static class Calculator
{
public static double Addition(double num1, double num2)
{
return (num1 + num2);
}
public static double Subtraction(double num1, double num2)
{
return (num1 - num2);
}
public static double Multiplication(double num1, double num2)
{
return (num1 * num2);
}
public static double Division(double num1, double num2)
{
return (num1 / num2);
}
}
}
Now, Add Testing Project
1: In Visual Studio 2019, Right-click on solution-> Add -> Project.
2: In the Create New Project dialog, select the Test ->xUnit Test Project (.Net Core) template, and click Next.
3: Enter the project name, and then click Create.
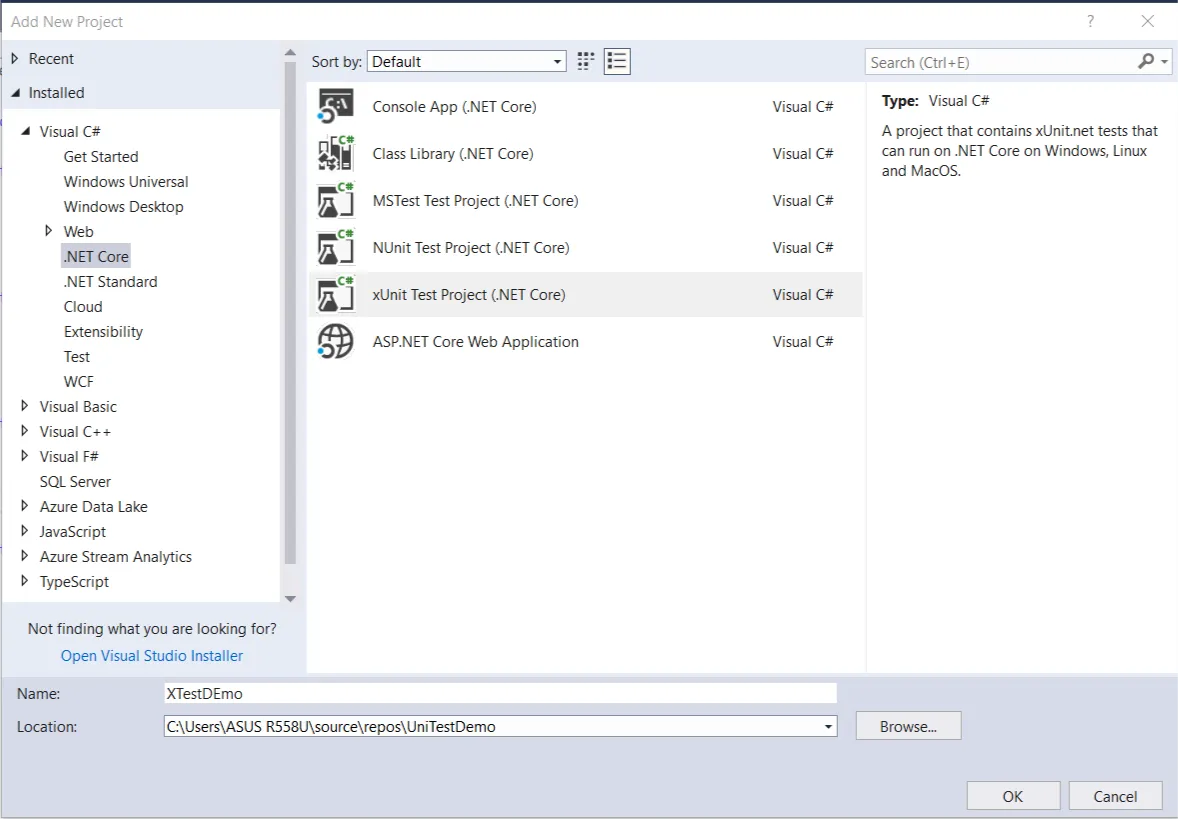
Figure 2 Add Testing Project
Step 5:Add Reference to your project
Right-click on your Testing project (XTestDEmo)->Add->reference
Figure 3 Add Reference to the testing project
Step 6:Now, Add one class in 'XTestDEmo' project named 'TestCalculator.cs'
In 'TestCalculator.cs' class we will write test case for method define in 'Calculator.cs'. These methods follow three-step: Arrange, Act, Assert.
The following class contains test cases for Calculator:
using System;
using UniTestDemo;
using Xunit;
namespace XTestDEmo
{
public class TestCalculator
{
[Fact]
public void Test_Addition()
{
// Arrange
var number1 = 4.9;
var number2 = 3.1;
var expectedValue = 8;
// Act
var sum = Calculator.Addition(number1, number2);
//Assert
Assert.Equal(expectedValue, sum, 1);
}
[Fact]
public void Task_Subtract_TwoNumber()
{
// Arrange
var number1 = 3.2;
var number2 = 1.5;
var expectedValue = 1.7;
// Act
var sub = Calculator.Subtraction(number1, number2);
//Assert
Assert.Equal(expectedValue, sub, 1);
}
[Fact]
public void Test_Multiplication()
{
// Arrange
var number1 = 2.5;
var number2 = 3.5;
var expectedValue = 8.75;
// Act
var mult = Calculator.Multiplication(number1, number2);
//Assert
Assert.Equal(expectedValue, mult, 2);
}
[Fact]
public void Test_division()
{
// Arrange
var number1 = 2.9;
var number2 = 3.1;
var expectedValue = 0.94; //Rounded value
// Act
var div = Calculator.Division(number1, number2);
//Assert
Assert.Equal(expectedValue, div, 2);
}
}
}
Step 7: Now, Run your test cases
For running all test cases open Test Explorer where you will find you're all test case. To run click on RunAll this will start executing test cases.
To open Test Explorer in visual studio Click on Test option->Window->Test Explorer.
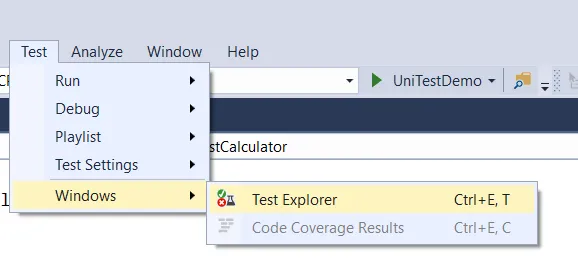
Figure 4 Opening Task Explorer
Once your test case runs successfully, the output screen will be shown as below.
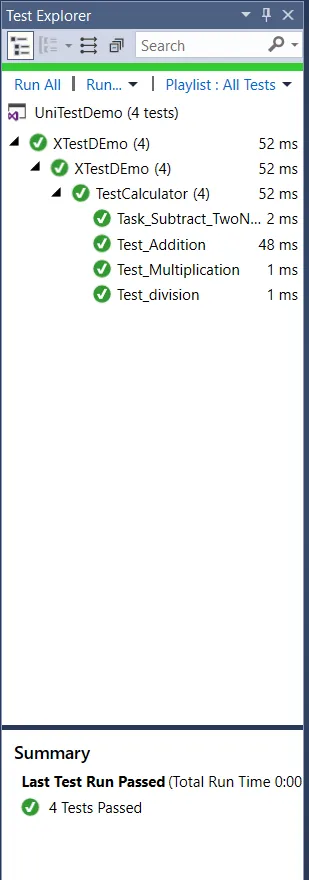
Figure 5 Output